Docker
Docker is used to build a container which is an enclosed space where our software can run without other software interfering with it. The docker software is basically a client-server software where the server runs a process called dockerd (Docker Daemon) and users can interact with it via a REST API from the terminal.Note: Even though REST concepts are something that we see in networking, docker follows the same except instead of urls it relies on file sockets

Workflow
Using the docker engine itself is simple. The workflow is shown below
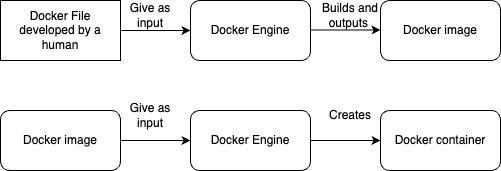
Usually for commonly used software like nginx, apache etc, the images are publicly available for us to just pull and use from registries that are present. Some of the registries are listed below
- Dockerhub
- Amazon Elastic Container Registry(ECR)
- Google Container Registry(GCR)
- Azure Container Registry(ACR)
- Quay (by Redhat)
- Github Packages Docker Registry
- Harbor
- JFrog Artifactory
- Sonatype Nexus Repository
But when it comes to our own software then we have to write the Dockerfile and then build the image and then share it
. Image names are usually in the form of image:tag or image:@digestBuilding your own custom image
To build a custom image create a file with the name Dockerfile in the directory where your project is. For example if we want to containerize a simple html file, here is how we would do it.
FROM nginx:alpine # nginx is the name of the image and alpine is the name of the tag RUN rm /usr/share/nginx/html/index.html # We are removing the default index.html file that comes in nginx servers COPY index.html /usr/share/nginx/html # And we are copying our own index.html to it EXPOSE 80 # We expose port 80 to let clients connect CMD ["nginx", "-g", "daemon off;"] # We tell the container to start the command nginx -g daemon off; ie start nginx in the foreground when a container is built
The difference between the RUN command and the CMD command is that RUN is used during building the image while CMD is for running commands when a container is created. Only the last CMD command will be considered
Now that we have this we can build an image out of this with the command docker build -t image_name .
. Here -t stands for tag name
docker build -t sample_image .
Once you do this, the building step happens which looks something like the below
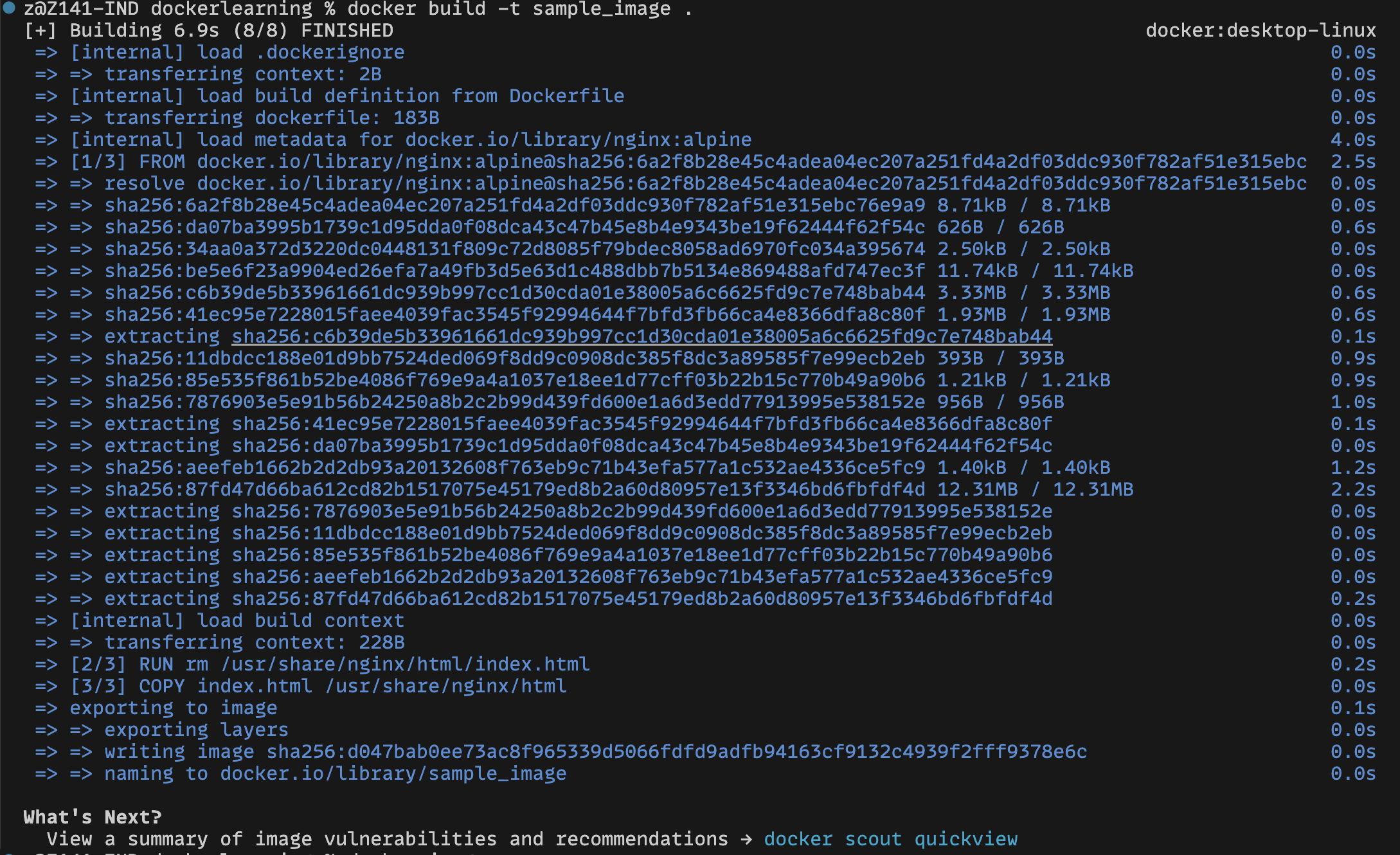
But there won't be any indication of an output file or anything anywhere. These images are stored in different places depending on the operating system, for Mac and Windows they are stored in a virtual machine file system that the Docker engine creates. It is not clear as of now whether this can be configured, it is however stored in /var/lib/docker in Linux though
To look at the list of images that are present in the computer, you can run docker images
which gives an output that looks like the following

You can delete these images by running docker rmi image_id1 image_id2
Note: It should be noted that a container needs like a running process for it to run. So for instance if you create a container with linux and a static html file, it will instantly stop. One way to keep it running is to run some dummy command like tail or something
For instance if we want to create a standalone docker image with nothing in it, except maybe a barebones OS we can simply put the following
FROM alpine # A small linux distro CMD ["tail", "-f", "/dev/null"] # A placeholder command so that the container does not terminate
Creating a container from an image and Deleting a container
To create a container we have two options:
- Create a container and run it immediately
- Create a container but run it later separately

The -d in the run command means we are starting the container in detached mode
Deletion of a Container
To delete a container we can just run the following command
docker rm container_idNote: Interestingly when we try to delete an image before deleting the container. Docker would throw an error stating that the image is being used by the container and would not allow us to do. I have to investigage why would it still need the image after the container has already been created.
Attaching and Detaching
When we create and run a container with the -d option, the container starts in a detached mode. Detached means that it will start in the background and control of the terminal will be given back to us
You should be able to attach to it by giving the following command
docker attach container_id
This will connect to the input/output and error of the docker container. But I do not yet know how to use this. To detach from it pressing Ctrl - Z works but online docs suggest pressing Ctrl P + Ctrl Q together which doesn't work for me
Executing commands inside Docker
A container basically contains a small filesystem where we can run commands. To send a command to execute inside a container we can run the following command
docker exec container_id commandExample:
docker exec container_id lsOR
docker exec container_id sh -c "cd /var"Note: Although in the above example running cd /var/ is of no use because it will execute and return. sh is the argument you provide to the docker exec command while the -c flag helps you provide an argument to the sh command inside the container
In case if you want to, just go inside the container's file system we can run it in as well
docker exec -it container_id sh
The -i flag is to be able to give interactive commands to the container. For example we could just run docker exec -i container_id sh
and it will just wait for you to give input and if you give a command like ls
and press enter it will display the output. It almost appears like a shell but without the user prompt or anything
The -t flag is to simulate a terminal, but usually it is recommended to do -it together, although it is unclear why as of now.
Docker Networks
Docker networks are merely an added layer of container (They call it networks though) where we can attach containers to the network and detach them from it. The idea is that all containers within a single network can easily talk to each other by the internal IP address that they are allotted inside the network
Commonly used commands with a network
Command | Description |
---|---|
docker create network network_name | Creates a network |
docker network ls | Lists all networks |
docker network connect network_name container_name | Attaches a container to a network |
docker network disconnect network_name container_name | Removes a container from the network |
docker network rm network_name | Deletes a network |
docker network inspect network_name | Inspects the network and shows the containers that are attached to it. Looks like JSON |